Optimal Merge Pattern Program In C
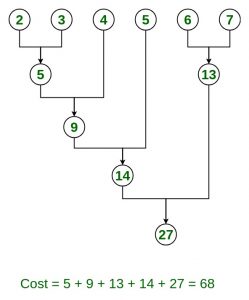
|
|
HomeSubmit CodeTop Code SearchLast Code SearchPrivacy PolicyLink to UsContact |
- Design and Analysis of Algorithms
Rite aprogram for optimal merge pattern.#include#includevoid mainclrscr;int i,k,a10,c10,n,l;cout. Pattern programs in C language, showing how to create various patterns of numbers and stars. The programs require nested loops (a loop inside another loop). A design of numerals, stars, or characters is a way of arranging these in some logical manner, or they may form a sequence.
- Basics of Algorithms
- Design Strategies
- Graph Theory
- Heap Algorithms
- Sorting Methods
- Complexity Theory
- DAA Useful Resources
- Selected Reading
Problem Statement
In job sequencing problem, the objective is to find a sequence of jobs, which is completed within their deadlines and gives maximum profit.
Solution
Let us consider, a set of n given jobs which are associated with deadlines and profit is earned, if a job is completed by its deadline. These jobs need to be ordered in such a way that there is maximum profit.
It may happen that all of the given jobs may not be completed within their deadlines.
Assume, deadline of ith job Ji is di and the profit received from this job is pi. Hence, the optimal solution of this algorithm is a feasible solution with maximum profit.
Thus, $D(i) > 0$ for $1 leqslant i leqslant n$.
Initially, these jobs are ordered according to profit, i.e. $p_{1} geqslant p_{2} geqslant p_{3} geqslant :.. : geqslant p_{n}$.
Analysis
In this algorithm, we are using two loops, one is within another. Hence, the complexity of this algorithm is $O(n^2)$.
Example
Let us consider a set of given jobs as shown in the following table. We have to find a sequence of jobs, which will be completed within their deadlines and will give maximum profit. Each job is associated with a deadline and profit.
Job | J1 | J2 | J3 | J4 | J5 |
---|---|---|---|---|---|
Deadline | 2 | 1 | 3 | 2 | 1 |
Profit | 60 | 100 | 20 | 40 | 20 |
Solution
To solve this problem, the given jobs are sorted according to their profit in a descending order. Hence, after sorting, the jobs are ordered as shown in the following table.
Job | J2 | J1 | J4 | J3 | J5 |
---|---|---|---|---|---|
Deadline | 1 | 2 | 2 | 3 | 1 |
Profit | 100 | 60 | 40 | 20 | 20 |
From this set of jobs, first we select J2, as it can be completed within its deadline and contributes maximum profit.
Next, J1 is selected as it gives more profit compared to J4.
In the next clock, J4 cannot be selected as its deadline is over, hence J3 is selected as it executes within its deadline.
The job J5 is discarded as it cannot be executed within its deadline.
Thus, the solution is the sequence of jobs (J2, J1, J3), which are being executed within their deadline and gives maximum profit.
Total profit of this sequence is 100 + 60 + 20 = 180.